250x250
반응형
Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 비트코인
- backtest
- 암호화폐
- probability
- Cloud
- backtrader
- TOEFL
- 퀀트
- AUTOSAR
- 파이썬
- it
- 블록체인
- 프로그래밍
- AWS
- can
- 백트레이더
- 백테스트
- python
- 토플
- Bitcoin
- 아마존 웹 서비스
- 오토사
- 자동매매
- 확률
- 자동차sw
- 토플 라이팅
- 클라우드
- toefl writing
- 개발자
- GeorgiaTech
Archives
- Today
- Total
Leo's Garage
Django #6 Make models more - 1 본문
728x90
반응형
자 이번에는 users model을 좀 더 업데이트 해보도록 하자.
from django.db import models
from django.contrib.auth.models import AbstractUser
# Create your models here.
class User(AbstractUser):
class GenderChoices(models.TextChoices):
MALE = ("male", "Male")
FEMALE = ("female", "Female")
class LanguageChoices(models.TextChoices):
KR = ("kr", "Korean")
EN = ("en", "English")
class CurrencyChoices(models.TextChoices):
WON = "won", "Korean Won"
USD = "usd", "Dollar"
first_name = models.CharField(max_length=150, editable=False)
last_name = models.CharField(max_length=150, editable=False)
avatar = models.ImageField(blank=True)
name = models.CharField(max_length=150, default="")
is_host = models.BooleanField(default=False)
gender = models.CharField(
max_length=10,
choices=GenderChoices.choices,
)
language = models.CharField(
max_length=2,
choices=LanguageChoices.choices,
)
currency = models.CharField(max_length=5, choices=CurrencyChoices.choices,)
자 새로운 property들을 추가 했다.
먼저 avatar라는 property를 생성했는데, models.CharField로 생성했다.
이 항목은 image에 대한 property라고 볼 수 있는데 즉, 프로필 이미지의 역할을 하게 된다.
blank = True는 비워둬도 괜찮다는 의미이다.
다음으로 gender, language, currency 항목을 추가하였는데, 이들은 char 형으로 생성하였고, choices라는 것을 통해서 사전에 class로 정의한 항목들을 선택할 수 있게 해준다.
자 이제 model.py를 변경하였으니 admin.py도 변경하도록 하자.
from django.contrib import admin
from django.contrib.auth.admin import UserAdmin
from .models import User
# Register your models here.
@admin.register(User)
class CustomUserAdmin(UserAdmin):
fieldsets = (
(
"Profile",
{"fields": ("avatar","username", "password", "name", "email", "is_host", "gender", "language","currency"),
"classes" :("wide",),
},
),
(
"Permission",
{
"fields": (
"is_active",
"is_staff",
"is_superuser",
"groups",
"user_permissions",
),
"classes":("collapse",),
},
),
(
"Important Dates", {
"fields": (
"last_login", "date_joined"
),
"classes":("collapse",),
}
),
)
list_display = ("username", "email", "name", "is_host")
fieldsets 내에 Profile fields에 신규로 생성한 property들을 추가하자.
그리고 나서 이전과 같이 명령어를 입력하자.
python manage.py makemigrations
python manage.py migrate
python manage.py createsuperuser
python manage.py runserver
위 명령어 수행 시, Pillow package를 설치하라고 에러 메시지가 나올 수 있다.
이는 ImageField 때문인데 이전에 우리는 poetry 환경에서 python 가상환경을 생성했기 때문에 아래와 같이 입력해준다.
poetry add Pillow
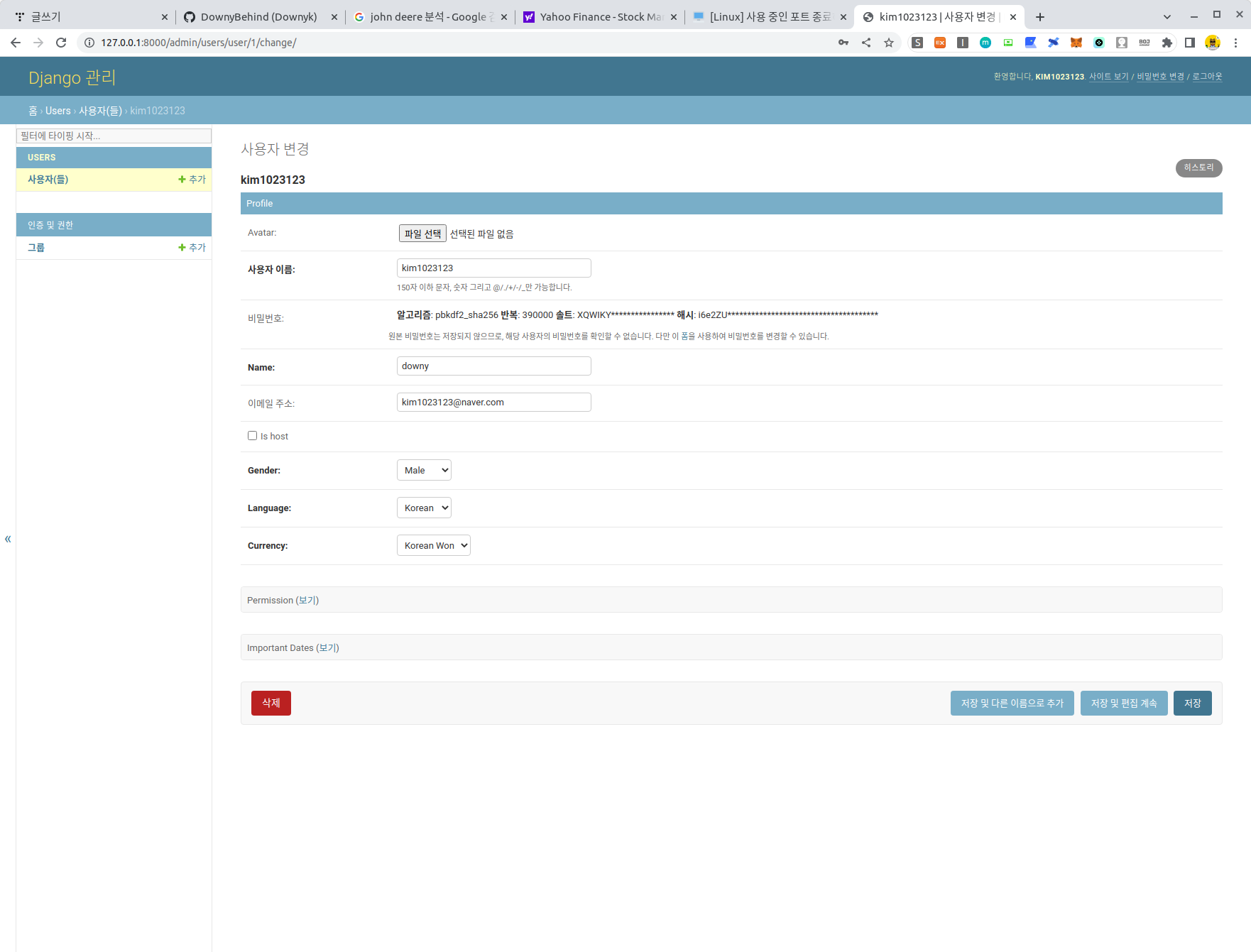
상기와 같이 user 데이터 변경 시, 추가한 property들이 들어가 있는 것을 알 수 있다.
728x90
반응형
'Study > Django' 카테고리의 다른 글
Django #5 Users App custom -2 (0) | 2023.01.02 |
---|---|
Django #4 Users App custom -1 (0) | 2022.12.30 |
Django #3 admin 기능 추가해보기 (0) | 2022.12.28 |
Django #2 app 만들기 (0) | 2022.12.28 |
Django #1 프로젝트 셋업 및 기본 동작 확인 (0) | 2022.12.27 |
Comments